Introduction
Python is one of the most popular programming languages. It is an interpreted high-level general-purpose language. Python is developed by Guido van Rossum in 1991. Its syntax is very beginner-friendly and easy to learn. That’s the reason why most people suggest python language to beginners as their first programming language to learn. In this post, we are going to discuss top python commands that can make your python learning journey easier.
In the Python programming language, commands basically refer to different functions or methods that we can execute on the python shell to work them as commands. According to the official documentation of Python, there are no “commands” in Python but we have different kinds of functions like input(), type(), len(), so on and so forth. So in this post, we’re going to use the terms commands and functions interchangeably. Now, let’s get started with the python commands list and discuss each command in detail.
Python Commands List
Basic Python Commands You Must Know
Here are the list of basic python commands.
Confused about your next job?
pip install command
pip is a package manager which is written in Python language. It is used to install and manage software packages. The pip install command is used to install any software package from an online repository of public packages, called the Python Package Index. To run this command in windows you need to open your Windows PowerShell and then use the following syntax to install any package.
Syntax: pip install package-name
Example:
print command
print command is used to print a message on the screen or other standard output device. The message can be a string or any other object. The print command can be used to print any type of object like integer, string, list, tuple, etc.
Syntax: print(object)
Example:
type command
type command is used to check the type or class of an object.
Syntax: type(object)
Example:
range command
range command is used to generate a sequence of integers starting from 0 by default to n where n is not included in the generated numbers. We use this command in for loops mostly.
Syntax: range(start, stop, step)
- start refers to the starting of range (Optional; 0 by default )
- stop refers to the number before which you want to stop (Mandatory)
- step refers to the increment count (Optional; 1 by default)
Example:
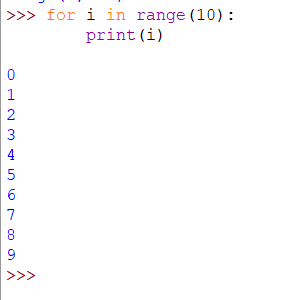
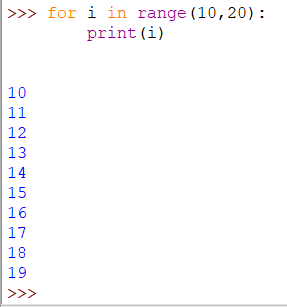
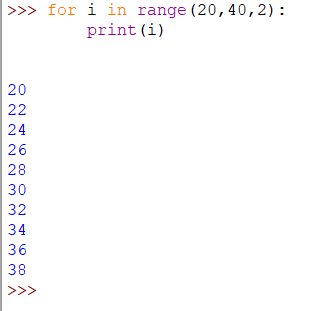
Note: If you provide two parameters to range() function, it will always consider them as (start,stop) and not as (stop,step).
round command
round command is used to round a number to a given precision in decimal digits. That means if you have so many digits after decimal in a float point number then you can use the round command to round off the specified number. You can mention how many digits you want after the decimal point.
Syntax: round(number, digits)
- number refers to the floating-point number.
- digits refer to the count of digits you want after the decimal point. (Optional; By default 0)
Example:
input command
input command is used to take input from the user. The flow of the program will be stopped until the user has entered any value. Whatever the user enters it will be converted into a string by the input function. If you want to take an integer as input then you have to convert it explicitly.
Syntax: input(message)
message refers to the text you want to display to the user. (Optional)
Example:
len command
len command or len() function is used to get the number of items in an object. If the object is a string then len() function returns the number of characters present in it. If the object is a list or tuple it will return the number of elements present in that list or tuple. len() gives an error if you try to pass an integer value to it.
Syntax: len(object)
object of which you want to find the length (Required)
Example:
Loop commands
In python, we have two primitive loop commands namely while and for. The while loop command is used to execute a set of statements as long as the given condition is true.
Syntax of while loop: while condition:
statements
update iterator
Example:
The for loop command is used to execute a set of statements by iterating over a sequence. This sequence can be a list, tuple, string, dictionary, etc.
Syntax of for loop: for x in sequence:
statements
Example:
Intermediate Python Commands
String Commands
In the python programming language, we have various string commands that we can use on string objects. These commands do not change the original string object, they just return a new object. Some of the most important string functions are:
isalnum(): It checks whether all the characters of a given string are alphanumeric or not. It returns a boolean value.
Syntax: stringname.isalnum()
Example:
capitalize()
capitalize() function changes the first character of the string to uppercase if it’s lowercase. If the first character is uppercase or an integer or any special character, it doesn’t do anything.
Syntax: stringname.capitalize()
Example:
find()
find() command is used to search for a substring in a string. It returns the index of the first occurrence of the substring if it is present otherwise it returns -1.
Syntax: string.find(substring)
- string refers to the string in which you want to search.
- substring refers to the value that you want to search.
Example:
- count(): count() function returns the count of occurrences of a substring in a string object.
Syntax: stringname.count(substring, start, end)
- stringname refers to the string in which you want to search.
- substring refers to the value whose count you want to find.
- start refers to that starting index within the string where search starts (Optional)
- end refers to that ending index within the string where the search ends (Optional)
Example:
center()
center command is used to align a string in the center, using a specified character (by default it is space) as the fill character.
Syntax: string.center(length, character)
- string is the string that you want to align in center
- length is the full length taken by the new string in which both sides will be filled by character and in the center, we will have the original string.
- character refers to the character used to fill the missing space on each side. Default is ” ” (space).
Example:
List Commands
Just like a string we have various commands for list objects as well. Lists are used to multiple elements in a single object. We can use lists to store elements of different data types. Some of the most important list methods are:
append(): append command is used to add an element at the end of the list.
Syntax: list.append(element)
- list is the list object in which you want to add an element
- element refers to the new item that you want to add to the list
Example:
copy()
copy() is used to create a new copy of the list object. It returns a new list object.
Syntax: list.copy()
Example:
insert()
insert command is used to add an element at a specified position in the list object. It takes two parameters position and element.
Syntax: listname.insert(position, element)
- position at which you want to insert a new element. If you give a position greater than the number of elements in the list, it will always insert at the end.
- element refers to the new element that needs to be added.
Example:
pop()
pop() method is used to remove an element from a specified position in the list. It returns the element after removing it from the list.
Syntax: listname.pop(position)
position from which you want to remove the element.
Example:
reverse()
reverse method reverses the order of all elements in the list. It modifies the original list object and it doesn’t return anything.
Syntax: list.reverse()
Example:
sort()
sort method is used to sort the elements of the list in ascending order by default.
Syntax: list.sort()
Example:
Tuple Commands
Tuple is a built-in data type that is used to store multiple elements in a single variable. Tuple objects are ordered, and immutable. There are only two built-in tuple methods which are as follows:
count(): count method is used to count the occurrences of an element in the tuple.
Syntax: tuple.count(element)
Example:
index()
This method is used to find the index of the first occurrence of an element. If the element is not found in the whole tuple then it will raise a ValueError.
Syntax: tuple.index(element)
- tuple is the tuple object in which you want to search an element
- element refers to the item that you wanna search
Example:
Advanced Python Commands List
Set Commands
Sets are also used to store multiple elements in a single object but they do not allow duplicate elements. Sets are unordered and unindexed. That means if you print all elements of a set they will get printed in random order. Once set is created you can not modify the elements but you can add new elements or can also remove existing elements. Now, let’s discuss some important set commands that python provides as built-in methods.
add(): add command is used to add a new element in the set.
Syntax: setname.add(element)
- setname is the name of that set variable in which you want to add a new element.
- element refers to the new item that you want to add.
Example:
clear()
clear removes all the elements of a set. It does not take any parameters.
Syntax: setname.clear()
Example:
discard()
discard is used to remove the specified element from the set. If the specified element is not found in the set it will not give an error.
Syntax: setname.discard(element)
- setname is the name of that set variable from which you want to remove an element.
- element refers to the element that you want to remove.
Example:
remove()
remove command is also used to remove a specified element from the set but it is different from discard because remove will give an error if the specified element is not found in the set.
Syntax: setname.remove(element)
- setname is the name of that set variable from which you want to remove an element.
- element refers to the element that you want to remove.
Example:
difference()
difference method is used to get a set that contains the difference of two sets. The difference of sets means it will have only those elements that are present in only one set and not in another set. Suppose we have two sets A and B. Set A has {1,2,3} and set B has {2, 4, 6}. Then the difference of A and B will be {1,3}.
Syntax: setA.difference(setB)
- Elements of setB will be removed from setA if present.
Example:
difference_update()
difference_update method is used to get a set of elements that are present in the first set and not common in both sets. That means it removes the elements that exist in both sets. It does not return a new set, it just removes common elements from the first set.
Syntax: setA.difference_update(setB)
- Elements that exist in both setA and setB will be removed from setA.
Example:
intersection()
intersection method returns a set having elements that exist in all the specified sets.
Syntax: set.intersection(set1, set2, … setn)
- Set of elements that exist in the set, set1, set2, … setn will be returned.
Example:
issubset()
issubset method checks whether all elements of set A are present in set B or not. It returns a boolean value.
Syntax: setA.issubset(setB)
Example:
symmetric_difference()
This method returns a set containing elements from both the sets except those that are common in both sets.
Syntax: setA.symmetric_difference(setB)
Example:
union()
union method returns a set containing all elements from both sets, except the duplicated ones.
Syntax: setA.union(setB)
Example:
Dictionary Commands
Dictionary is a built-in type in Python. It is used to store key-value pairs. It is ordered, modifiable, and does not allow duplicate keys. That means in a dictionary we can not add two pairs having the same value of keys. Python provides a set of built-in methods that we can use on dictionary objects.
fromkeys(): fromkeys() method is used to generate a dictionary with specified keys and a specified value.
Syntax: dict.fromkeys(keys, value)
- keys is the tuple or list of key elements.
- value refers to the value which would be paired with all the specified keys.
Example:
get()
get method is used to get a value of the specified key. If a key is not found in the dictionary it will return nothing unless we specify something in the parameters.
Syntax: dictionary.get(key, value)
- dictionary is the name of the dictionary object in which you want to search.
- key refers to the key that you want to search in the dictionary.
- value is the value that would be returned if the key is not found in the dictionary.
Example:
items()
items method is used to display the dictionary elements. It will display all the key-value pairs present in the dictionary. It returns a view object which will contain all the key-value pairs as tuples in a list. It does not take any parameters.
Syntax: dictionary.items()
Example:
keys()
keys method is used to get all the keys present in the dictionary. It returns a view object containing all keys of the dictionary as a list. It does not take any parameters.
Syntax: dictionary.keys()
Example:
values()
values method is used to get all the values present in the dictionary. It returns a view object containing all values of the dictionary as a list. It does not take any parameters.
Syntax: dictionary.values()
Example:
pop()
pop method is used to remove a key-value pair from the dictionary by specifying the key. It returns the value of the key-value pair that is need to be removed.
Syntax: dictionary.pop(key)
- key refers to the key of the pair that you want to remove from the dictionary.
Example:
popitem()
popitem command is used to remove the last inserted pair from the dictionary. It does not take any parameters. It returns the removed pair as a tuple.
Syntax: dictionary.popitem()
Example:
setdefault()
setdefault method is used to get the value of a specified key. If the key does not exist it will insert the key with the value passed as a parameter. If you don’t specify any value it will insert the key with value None.
Syntax: dictionary.setdefault(key, value)
Example:
Conclusion
In this post, we’ve discussed the top python commands that every Python programmer should learn. You should definitely try each and every command on your own. Also, try to experiment with random input parameters to see the behavior of commands. Python commands are easy to use, easy to write, and easy to learn. You don’t need to remember all the commands but you should be familiar with what all commands provide as functionality.
Frequently Asked Questions
Q. What is Python 3 command?
A. Python 3 commands are released in 2008. These commands are introduced with Python 2 features and they are compatible with Python 2 as well. Python 3 commands are more intuitive to programmers and more precise while providing the result.
Q. How do you use Python commands?
A. To use or run Python commands you can use python shell. Python Shell is the python interpreter which is used to execute simple Python programs and to run python commands. It just provides a quick way to execute commands without creating any file.
Q. What are some common Python commands?
A. Some common Python commands are input, print, range, round, pip install, len, sort, loop commands like for and while so on and so forth.
Q. What are magic commands in Python?
A. Magic commands are shortcuts or enhancements over the usual Python syntax. These commands are designed to facilitate routine tasks. They enable us to easily control the behavior of the IPython system and solve various common problems in standard data analysis, for example, running an external script or calculating the execution time of a piece of code.
Additional resources
Free Python Course
Practice Coding
Python MCQ
Python Interview Questions
PHP Vs Python
Python Developer Resume
Python Applications
Python Developer Skills